How to Plot Histogram from a Vector in ggplot2
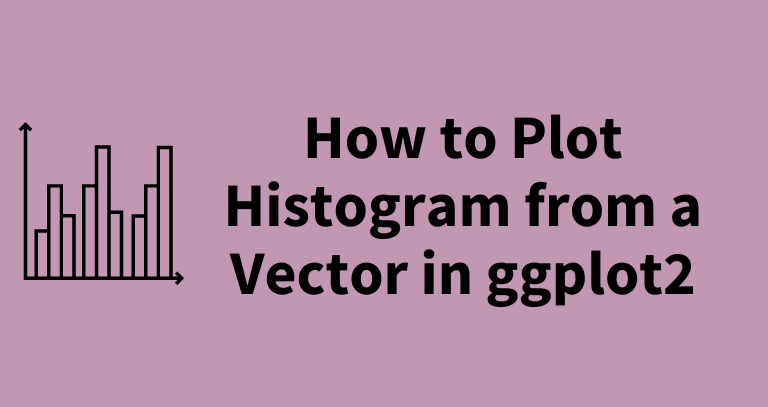
You can use the geom_histogram()
function in ggplot2 to create a histogram in R.
Generally, the ggplot()
accepts the data frame to create the histogram. But in this article,
we will cover how to use the numeric vector to create a histogram using ggplot()
and
geom_histogram()
.
The following examples demonstrate how to use numeric vectors to create a histogram in ggplot2.
1 Convert vector to data frame
Create a random numeric vector using the built-in rnorm()
function,
set.seed(1234)
num = rnorm(100, mean = 3, sd = 1)
Create a histogram using ggplot()
and geom_histogram()
,
# load package
library(ggplot2)
# create histogram using ggplot
ggplot(data.frame(num), aes(num)) + geom_histogram()
In this example, we have converted a numeric vector into a data frame using data.frame()
function and passed it to the
ggplot()
.
2 Construct aesthetic mappings
You can also construct aesthetic mappings (aes()
) as individual layer for the vector input instead of
passing it in a ggplot()
function.
Create a random numeric vector using the built-in rnorm()
function,
set.seed(1234)
num = rnorm(100, mean = 3, sd = 1)
Create a histogram using aes()
as individual layer for the vector input,
# load package
library(ggplot2)
# create histogram using ggplot
ggplot() + aes(num) + geom_histogram()
In this example, we have used the aes()
function as an individual layer for the vector input to create a histogram using ggplot()
.
3 Use aesthetic mappings in geom_histogram()
You can also construct aesthetic mappings (aes()
) inside geom_histogram()
for the vector input for
a creating histogram using ggplot()
function.
Create a random numeric vector using the built-in rnorm()
function,
set.seed(1234)
num = rnorm(100, mean = 3, sd = 1)
Create a histogram using aes()
within geom_histogram()
for the vector input,
# load package
library(ggplot2)
# create histogram using ggplot
ggplot() + geom_histogram(aes(num))
In this example, we have used the aes()
function inside geom_histogram()
for the vector input to create a histogram using ggplot()
.