Python: Replace Value at Specific Index in List and Array
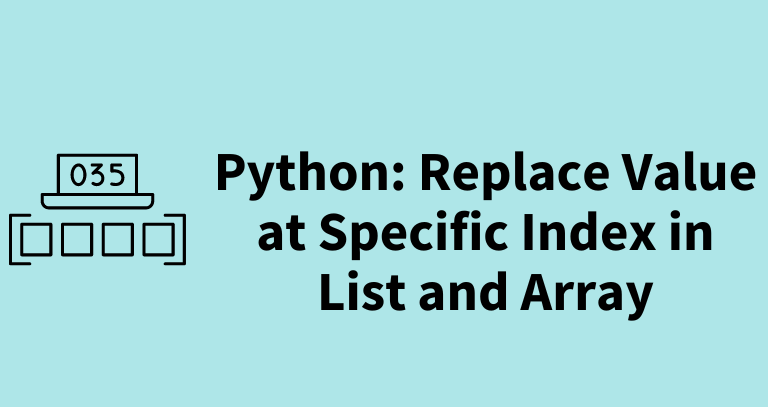
Contents
You can replace a value at a specific index in a list in Python by using direct indexing.
The basic syntax for replacing a value at a specific index using direct indexing:
# replacing the value at index 3 (remember in python index start at 0)
ex_list[3] = 100
Tip
In Python, lists and numpy arrays are mutable and you can modify list or array elements directly
by updating the indexes.
The following examples demonstrate replacing a value at a specific index in a list or array in Python.
Replace value at a specific index in list
Create an example list,
ex_list = [10, 20, 30, 40, 50, 60]
Replace a value 30 (which is at index 2) with 300,
ex_list[2] = 300
print(ex_list)
# ouput
[10, 20, 300, 40, 50, 60]
You can see that we replaced the value 30 at index 2 with 300 in a list in Python.
Replace value at a specific index in numpy array
Create an example array,
# import package
import numpy as np
ex_array = np.array([10, 20, 30, 40, 50, 60])
Replace a value 30 (which is at index 2) with 300 in numpy array,
ex_array[2] = 300
print(ex_array)
# output
[ 10 20 300 40 50 60]
You can see that we replaced the value 30 at index 2 with 300 in a numpy array in Python.