Find and Replace Values in List in Python
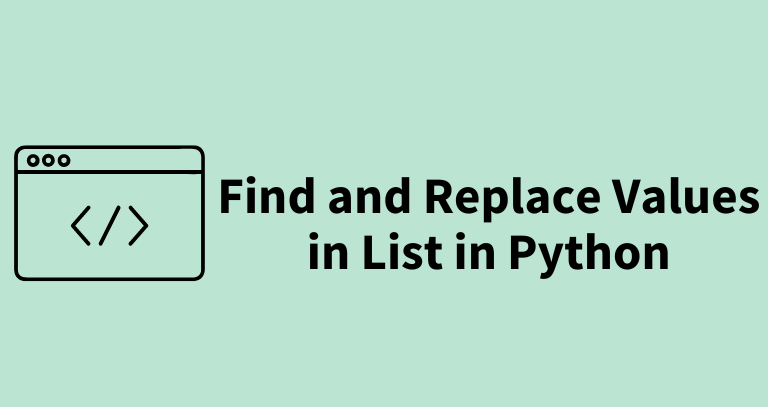
You can find and replace string values in a list using a list comprehension or map()
function in Python.
Method 1: list comprehension
new_list = [s.replace('old_string', 'new_string') for s in input_list]
Method 2: map()
function
new_list = map(lambda s: str.replace(s, 'old_string', 'new_string'), input_list)
The following examples demonstrate how to use list comprehension and map()
functions to replace string values
in a list in Python.
Example 1: find and replace string using list comprehension
Create a sample list,
input_list = ['country', 'city', 'village', 'county']
If you want to replace a specific string in the above list, you can use the list comprehension method.
For example, if you want to replace the village
string with the city
string, you can use the list comprehension
method as below.
new_list = [s.replace('village', 'city') for s in input_list]
print(new_list)
# output
['country', 'city', 'city', 'county']
In the above code, list comprehension is used to iterate over each item in the input list and replace the string
using the replace()
function when there is a match.
In addition to string replacement, you can also use list comprehension to replace the characters in the string.
input_list = ['country;', 'city;', 'village', 'county']
# replace semicolon(;) with colon (:)
new_list = [s.replace(';', ':') for s in input_list]
print(new_list)
# output
['country:', 'city:', 'village', 'county']
Example 2: find and replace string using map()
Create a sample list,
input_list = ['country', 'city', 'village', 'county']
If you want to replace a specific string in the above list, you can use the map()
function.
For example, if you want to replace the village
string with the city
string, you can use the map()
function as below.
new_list = list(map(lambda s: str.replace(s, 'village', 'city'), input_list))
print(new_list)
# output
['country', 'city', 'city', 'county']
In the above code, the lambda
function checks if each item in the list matches to village
and replaces it with the
city
string. Additionally, the list()
function is used to convert the map object into a list.